Unlocking the Secrets: Crafting Interactive Websites with PHP and MySQL
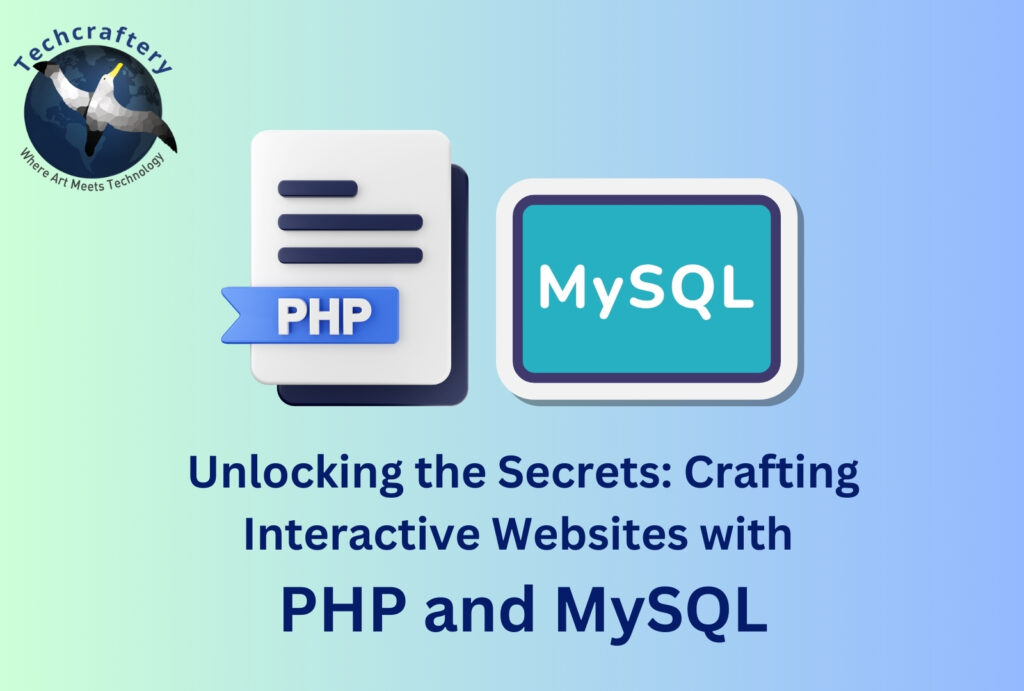
Creating dynamic and interactive websites is essential in today’s digital landscape. Websites that engage users and provide a seamless experience are powered by robust backend technologies. Among these technologies, PHP (Hypertext Preprocessor) and MySQL (Structured Query Language) stand out as powerful tools for web development. This guide will walk you through the essentials of using PHP and MySQL to craft interactive websites.
1. Introduction to PHP and MySQL
PHP is a server-side scripting language designed for web development but also used as a general-purpose programming language. MySQL, on the other hand, is a relational database management system that allows you to store, manage, and retrieve data efficiently.
Together, PHP and MySQL enable developers to create dynamic websites that can interact with users, process data, and present information in a meaningful way.
2. Setting Up Your Development Environment
Before diving into coding, it’s essential to set up your development environment.
Installing XAMPP
XAMPP is a free and open-source cross-platform web server solution stack package. It is easy to install and allows you to run PHP and MySQL on your local machine.
- Download XAMPP: Visit the XAMPP website and download the latest version for your operating system.
- Install XAMPP: Follow the installation instructions. Choose the components you want to install, including Apache and MySQL.
- Start the XAMPP Control Panel: Once installed, open the XAMPP Control Panel and start Apache and MySQL services.
Setting Up PHP and MySQL
After installing XAMPP, you can access PHP and MySQL through your browser.
- To access PHP, open your browser and go to
http://localhost/dashboard/
. - To manage MySQL databases, click on phpMyAdmin from the XAMPP dashboard.
3. Understanding PHP Basics
Syntax and Variables
PHP code is written between <?php
and ?>
tags. Here’s a simple example:
phpCopy code<?php
$greeting = "Hello, World!";
echo $greeting;
?>
In this example, $greeting
is a variable that stores a string. The echo
statement outputs the value of the variable.
Control Structures
Control structures such as if-else statements and loops allow you to control the flow of your program.
If-Else Example:
phpCopy code<?php
$age = 20;
if ($age >= 18) {
echo "You are an adult.";
} else {
echo "You are a minor.";
}
?>
For Loop Example:
phpCopy code<?php
for ($i = 1; $i <= 5; $i++) {
echo "Number: $i<br>";
}
?>
Functions
Functions are reusable blocks of code. Here’s how to create a simple function in PHP:
phpCopy code<?php
function add($a, $b) {
return $a + $b;
}
echo add(5, 10); // Outputs: 15
?>
4. Introduction to MySQL
What is MySQL?
MySQL is a powerful open-source relational database management system. It is widely used for storing and retrieving data in web applications.
Database Basics
A database is a structured collection of data. In MySQL, data is organized into tables, which consist of rows and columns.
Creating a Database:
To create a new database, you can use the following SQL command in phpMyAdmin:
sqlCopy codeCREATE DATABASE my_database;
5. Connecting PHP to MySQL
To interact with a MySQL database using PHP, you need to establish a connection.
Using MySQLi
MySQLi (MySQL Improved) is a PHP extension that provides a way to access MySQL databases.
phpCopy code<?php
$conn = new mysqli("localhost", "username", "password", "my_database");
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
?>
Using PDO
PDO (PHP Data Objects) is a database access layer that provides a uniform method for access to multiple databases.
phpCopy code<?php
try {
$conn = new PDO("mysql:host=localhost;dbname=my_database", "username", "password");
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully";
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
6. CRUD Operations with PHP and MySQL
CRUD stands for Create, Read, Update, and Delete. These are the four basic functions of persistent storage.
Create
To insert data into the database:
phpCopy code<?php
$sql = "INSERT INTO users (username, email) VALUES ('john_doe', 'john@example.com')";
if ($conn->query($sql) === TRUE) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
?>
Read
To retrieve data from the database:
phpCopy code<?php
$sql = "SELECT * FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "id: " . $row["id"]. " - Name: " . $row["username"]. " - Email: " . $row["email"]. "<br>";
}
} else {
echo "0 results";
}
?>
Update
To update existing data:
phpCopy code<?php
$sql = "UPDATE users SET email='john_new@example.com' WHERE username='john_doe'";
if ($conn->query($sql) === TRUE) {
echo "Record updated successfully";
} else {
echo "Error updating record: " . $conn->error;
}
?>
Delete
To delete data:
phpCopy code<?php
$sql = "DELETE FROM users WHERE username='john_doe'";
if ($conn->query($sql) === TRUE) {
echo "Record deleted successfully";
} else {
echo "Error deleting record: " . $conn->error;
}
?>
7. Building a Dynamic Website
Project Overview
Let’s build a simple dynamic website where users can register and view their profiles. This project will utilize the CRUD operations we’ve learned.
Designing the Database
First, create a database named user_management
and a table named users
with the following structure:
sqlCopy codeCREATE TABLE users (
id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(30) NOT NULL,
email VARCHAR(50),
reg_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP
);
Creating the Frontend
- HTML Form for Registration:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>User Registration</title>
</head>
<body>
<h2>Register</h2>
<form action="register.php" method="POST">
Username: <input type="text" name="username" required><br>
Email: <input type="email" name="email" required><br>
<input type="submit" value="Register">
</form>
</body>
</html>
- Display User Profiles:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>User Profiles</title>
</head>
<body>
<h2>User Profiles</h2>
<?php
// Include database connection
include 'db_connection.php';
$sql = "SELECT * FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "ID: " . $row["id"]. " - Name: " . $row["username"]. " - Email: " . $row["email"]. "<br>";
}
} else {
echo "0 results";
}
?>
</body>
</html>
Developing the Backend
- Register User (register.php):
phpCopy code<?php
include 'db_connection.php';
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$username = $_POST['username'];
$email = $_POST['email'];
$sql = "INSERT INTO users (username, email) VALUES ('$username', '$email')";
if ($conn->query($sql) === TRUE) {
echo "Registration successful!";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
}
?>
8. Enhancing User Experience
Input Validation
It’s important to validate user input to prevent errors and enhance security. Use PHP’s built-in functions to sanitize inputs:
phpCopy code$username = htmlspecialchars($_POST['username']);
$email = filter_var($_POST['email'], FILTER_SANITIZE_EMAIL);
Error Handling
Implement error handling to manage unexpected situations. Use try-catch blocks in PDO or check for errors in MySQLi.
Security Measures
To secure your application:
- Use prepared statements to prevent SQL injection.
- Implement user authentication for sensitive actions.
- Regularly update your PHP and MySQL versions.